What is CSR and SSR
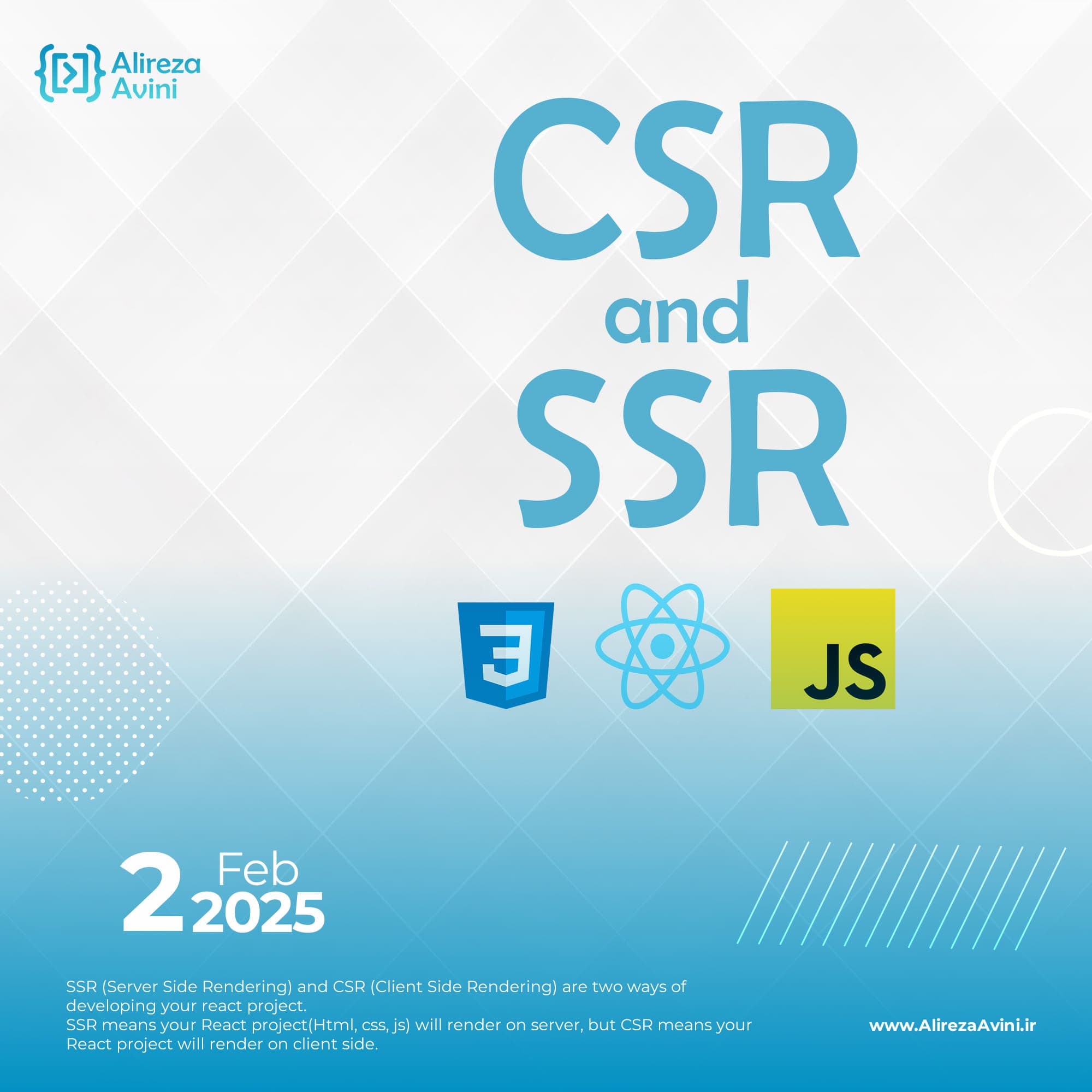
When learning React.js, you’ll encounter two key rendering strategies: SSR (Server-Side Rendering) and CSR (Client-Side Rendering). These approaches impact how your application loads, performs, and ranks on search engines. Let’s dive into what they are, how they differ, and when to use each.
Client Side Rendering (CSR):
Traditionally, all single-page web applications were rendered on the client-side. In a client side rendering process, the role of a server is limited to storing the client-side code of the application and data and transferring them to the browser when requested.
The user’s browser downloads the JavaScript bundle, executes it, and uses it to render the UI and add interactivity. This process happens entirely on the client side.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>CSR</title>
</head>
<body>
<div id="root"><!-- blank --></div>
<script src="/bundle.js"></script>
</body>
</html>
Pros of Client Side rendering:
Client-side rendered sites are faster to navigate after the initial load because subsequent page transitions happen without full page reloads. Only the necessary data is fetched, and the UI updates dynamically using JavaScript.
Cons of CSR
CSR can negatively impact SEO because search engine bots may struggle to crawl and index content that is dynamically rendered by JavaScript. While modern search engines like Google have improved at rendering JavaScript, CSR can still lead to slower indexing and poorer SEO performance compared to SSR.
Server-Side Rendering (SSR)
In Server-Side Rendering, the server pre-renders the HTML for each page, including the necessary data, and sends it to the browser. This allows the browser to display the content immediately, even before JavaScript is fully loaded.
An example of HTML file, containing a simple static content, that browser received with server-side rendering. All HTML elements inside the root element were rendered on the server:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SSR</title>
</head>
<body>
<div id="root">
<div className="container">
<h2>Server Side Rendering</h2>
<p>This content was rendered on Server Side</p>
</div>
</div>
<script src="/bundle.js"></script>
</body>
</html>
Pros of SSR
Reduced latency: Since the server pre-renders the page with the necessary data, the browser receives a fully-rendered page without waiting for additional API calls. This is especially beneficial for users with slower internet connections or those far from the server.
Cons of SSR
Server-Side Rendering can be resource-intensive because the server must handle rendering for every request. This increases server load and costs, especially for high-traffic applications. Additionally, navigating between pages may require full page reloads, which can feel slower compared to CSR’s dynamic updates.